UITextView코드로 작성하기
1. TextView 생성하기
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
private let commentTextView: UITextView = {
let textView = UITextView()
//Design
textView.backgroundColor = .white
textView.textColor = .black
textView.layer.borderColor = UIColor.black.cgColor
textView.layer.borderWidth = 1
textView.text = "텍스트를 입력해주세요"
textView.isScrollEnabled = true
//Constraints
textView.widthAnchor.constraint(equalToConstant: 200).isActive = true
textView.heightAnchor.constraint(equalToConstant: 50).isActive = true
textView.translatesAutoresizingMaskIntoConstraints = false
return textView
}()
|
cs |
[TextView 생성하기]
textView.isScrollEnabled를 true로 해주어야 constraints 설정해 둔 것과 충돌이 일어나지 않았다.
textView.isScrollEnabled는 입력한 텍스트가 길어지면 자동으로 스크롤되는 기능이다.
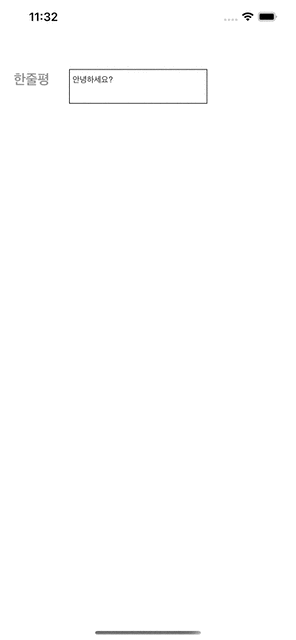
False로 설정하면 자동으로 스크롤이 안되어 텍스트 뷰 크기에 넘어가는 글은 보이지 않게된다.
뿐만 아니라 constraints 관련 에러가 발생했다. (Constraints를 따로 설정하지 않았다면 해당 에러는 없겠지만 여전히 많은 텍스트를 작성할 수는 없을 것이다.)
EditViewControllerTest[50912:1784601] [LayoutConstraints] Unable to simultaneously satisfy constraints.
Probably at least one of the constraints in the following list is one you don't want.
Try this:
(1) look at each constraint and try to figure out which you don't expect;
(2) find the code that added the unwanted constraint or constraints and fix it.
(
"<NSLayoutConstraint:0x60000119ddb0 UITextView:0x7fd443814800'\Ud14d\Uc2a4\Ud2b8\Ub97c \Uc785\Ub825\Ud574\Uc8fc\Uc138\Uc694'.width == 200 (active)>",
"<NSLayoutConstraint:0x6000011a64e0 'fittingSizeHTarget' UITextView:0x7fd443814800'\Ud14d\Uc2a4\Ud2b8\Ub97c \Uc785\Ub825\Ud574\Uc8fc\Uc138\Uc694'.width == 0 (active)>"
)
2. Constraints 에러
위와 같은 에러는 중복되는 constraints가 있을 경우에 발생하는 에러인 것 같은데,
이상하게 TextView가 들어간 경우에 width에서 가장 많이 발생했었다.
1
2
3
4
5
6
7
8
9
10
|
func commentStackViewLayout() {
commentStackView.addArrangedSubview(commentLabel)
commentStackView.addArrangedSubview(commentTextView)
commentStackView.topAnchor.constraint(equalTo: view.topAnchor, constant: 100).isActive = true
commentStackView.leftAnchor.constraint(equalTo: view.leftAnchor, constant: 20).isActive = true
// commentStackView.widthAnchor.constraint(equalToConstant: 250).isActive = true
// commentStackView.heightAnchor.constraint(equalToConstant: 60).isActive = true
}
|
cs |
[TextView 생성하기] 코드에서 생성된 commentTextView를 stackview에 넣고, 해당 stackview의 레이아웃을 정해 준 코드이다.
계속해서 constraints에러가 발생했었는데, comment out 처리된 7번 라인의 코드가 문제였다. 7번 라인을 비활성화하니 더 이상 에러가 나오지 않았다. 스택뷰의 widthAnchor와 textView의 widthAnchor 둘 다 설정되어 있어서 그런 문제가 일어나는 것 같았다.
하지만 아직 이해가 되지 않는 건, heightAnchor도 둘 다 설정되어 있을 때는 문제가 생기지 않는다는 점이다.
코드에 문제가 있는 것이겠지만 아직 정확히 어떤 부분이 문제인지 찾아내지 못했다.
3. UI 관련 기능들
1) 셀 간 라인 없애기
UITableView는 따로 설정을 하지 않으면 기본적으로 셀 간 구분을 위해 한 줄의 라인이 나온다.
이 라인을 separator 라고 부르는데, separator를 원하지 않는다면 없앨 수도 있다.
1
|
tableView.separatorStyle = .none
|
cs |
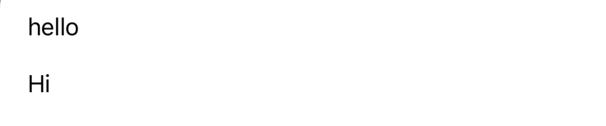
그러면 이렇게 깔끔하게 라인이 사라진 것을 확인할 수 있다.
[참고]
https://stackoverflow.com/questions/26653883/delete-lines-between-uitableviewcells-in-uitableview